My recent blog post on creating a Facebook Conversions API (aka CAPI) using Pipedream has been quite a success. Released in February, it’s already ranking as our 4th most popular blog post on my blog.
One of the most frequent questions I get about this post is how come I didn’t choose Integromat or Zapier instead of Pipedream. While I’m a big fan of these two platforms (Integromat slightly more), Pipedream simply offers better pricing for scale. This is very lucrative if you’re switching your entire pixel tracking to server-side (i.e. including Pageviews), which can easily add up to tens of thousands of events.
At Zapier or Integromat’s pricing, this becomes a major expense. So Pipedream was my go to for this.
But some advertisers want a simpler solution (though in all fairness I think it’s simple enough), so I figured I’d write up a quick guide for implementing Facebook’s Conversions API using Zapier.
Important note
This guide will help you build a CAPI solution that is utilizing the key concepts of the API but is built as a client-side solution. This means you won’t get the full benefits of the CAPI (mainly overcoming ad blockers) but can expect an increase of 5-10% in the number of events tracked, resulting in larger audiences to target in your campaigns (e.g. more users recorded that added to their carts).
Facebook even released this case study with “Love your Melon” which showed a 17% increase in attributed revenue after switching to the Conversions API.
When is Zapier the right choice for you?
- You’re lazy – No need to be ashamed of it. You have other, more important, stuff to handle so you’re willing to pay the premium
- You’re tracking only few events – In many cases, advertisers don’t need each and every event tracked using the CAPI solution, making the overall sum events sent smaller
Important note:
To implement the CAPI solution via Zapier you are required to have a paid Zapier account (as the free account doesn’t have the Webhook app (Premium App)
Benefits of using Zapier
- Simpler set up – no need to extract your Pixel or Token, just click on your ad account and you’re all set
- No code – While Pipedream uses a ready made code snippet, you still need to make minor changes to it
Step 1 – Create a Zap & Trigger
In your Zapier account, create a New Zap. The Zap’s trigger should be set to “Webhooks by Zapier” and the trigger event to “Catch hook”.
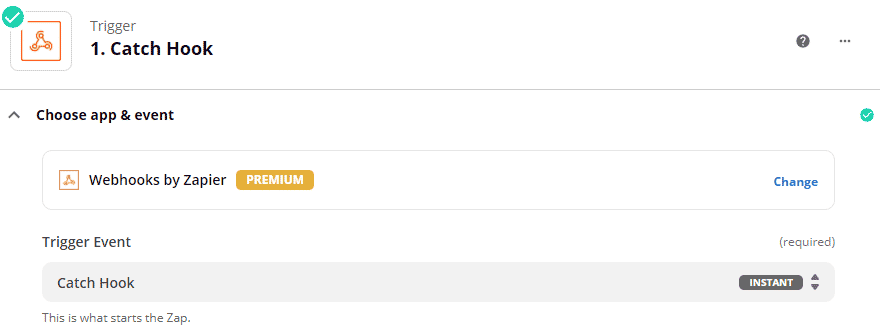
After clicking Continue, you will receive the Custom Webhook URL, e.g. https://hooks.zapier.com/hooks/catch/123456789/abc123456/ Copy this URL and move to the “Test trigger” step.
Sending a test trigger
The test trigger will now determine the structure of the data sent into Zapier, so it’s important to get this correct.
- Open a new tab with Request Bin
- Paste the Webhook URL in the top box
- Change the call type from GET to POST
- Under the Content tab, select JSON (application/json) from the drop down
- Paste the JSON values bellow in the text box
- Hit Send
{
"event": "Purchase",
"currency": "USD",
"value": 30.00,
"fbp": "fbp123",
"fbclid": "fbc456",
"user_agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.77 Safari/537.36 Edg/91.0.864.37",
"url": "https://fixel.ai/"
}
Important note:
The above JSON snippet represents a Purchase event. You can change it freely to use any other Facebook Pixel event you need (e.g. Lead or Add to Cart).
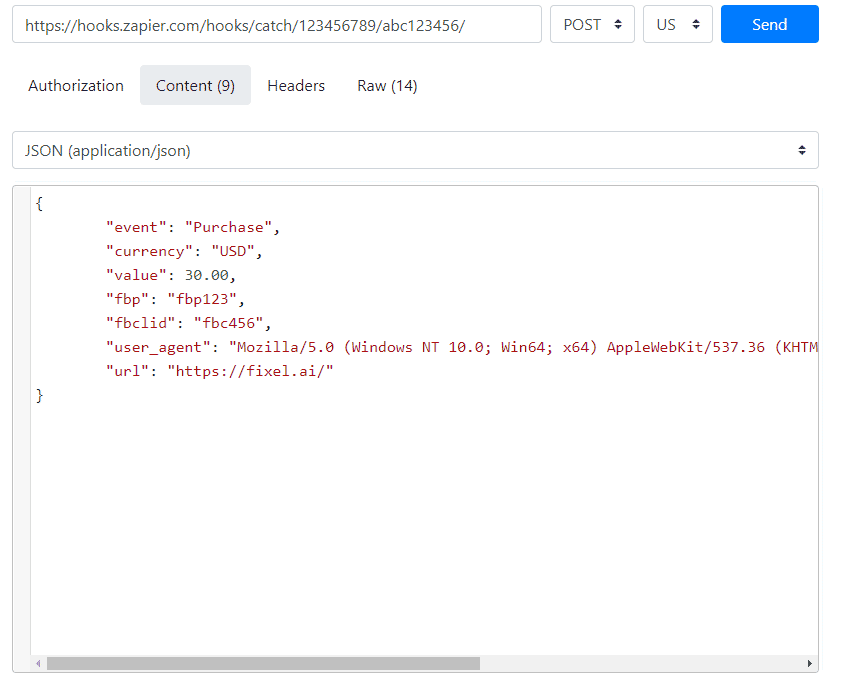
Once received in Zapier, you will have these values available to continue creating your Zap.
Step 2 – Create an Action
Now that we have the webhook dummy data available we can create a new action to pass that data to Facebook. Immediately under the Trigger, create a new Action with the app “Facebook Conversion”. Under “Action Event”, you can either choose a specific event (Purchase, Lead) or Other, which gives you the option to trigger other events (e.g. Add to Cart or Start Trial).
Pro tip:
You can use the “Send Other Event” option to create a dynamic action (i.e. a single webhook dynamically triggers a specific event type)
For sake of simplicity, let’s select the Purchase event.
Set the Action Source to Website.
Next, you will need to select the relevant Facebook Ad Account/Business Manager and then the relevant pixel for which the event is triggered.
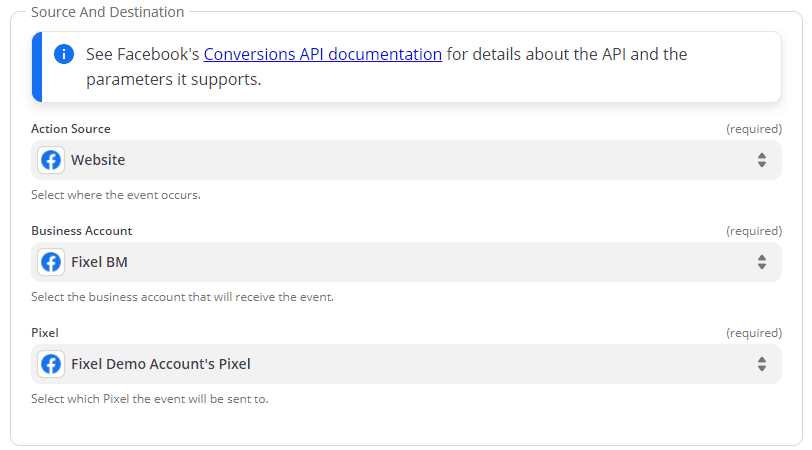
Under the Event Info section, set the Event Source URL to the URL parameter received in the webhook.
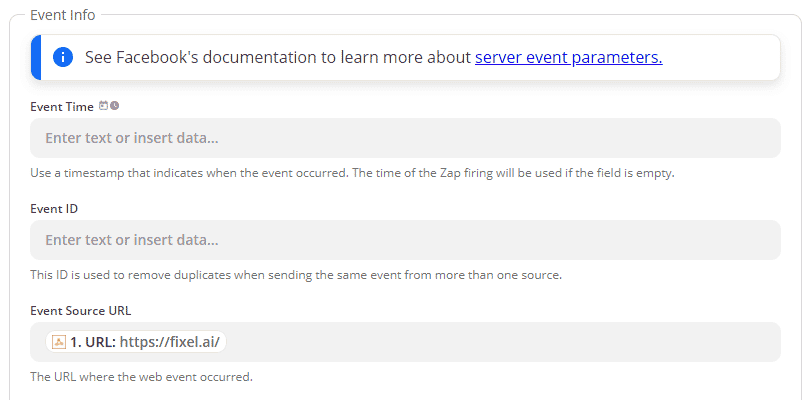
Under the Customer Information section, set the following values:
- Client User Agent -> User Agent
- Click ID -> Fbclid
- Browser ID -> Fbp
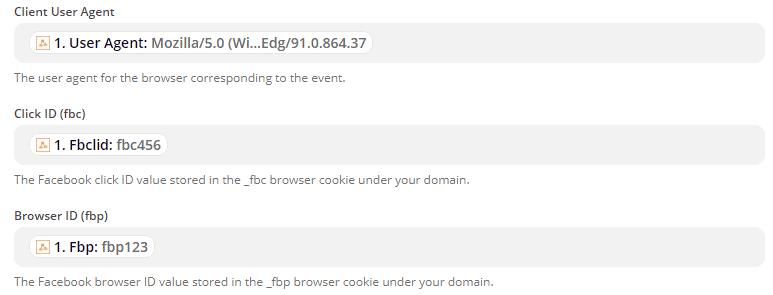
Under the Custom Data section, set the following values:
- Value -> Value
- Currency -> Currency
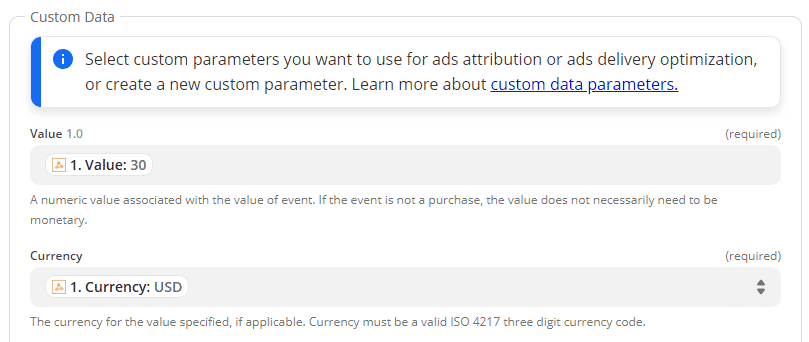
Pro tip:
You can send additional parameters in your request to provide more data about the event. Note that events must use the required parameters as defined per each event type.
After the mapping is finished, you can now test the event. You can also add the Test Event Code to the events sent to see them in real-time in your Facebook Event Manager.
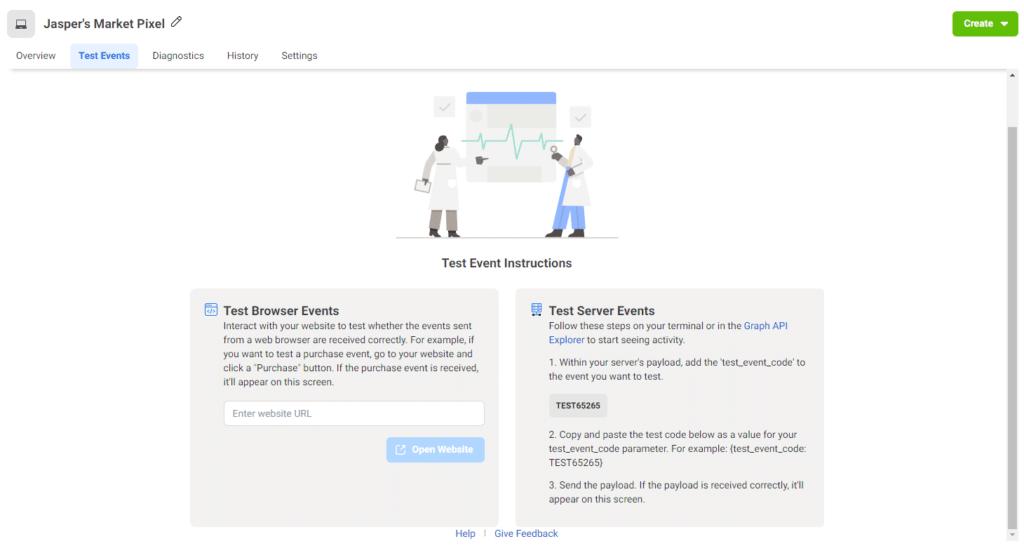
Step 3 – Adding the client-side script
After completing the Zapier setup, we now need to hop over to Google Tag Manager (GTM) to handle the events on happening on the website. Without completing this, no events will be sent to Zapier. In GTM, create a new Custom HTML tag and paste in the code below.
<script>
function CAPI(event, eventData) {
var fbp = document.cookie.split(';').filter(function (c) {
return c.includes('_fbp=');
}).map(function (c) {
return c.split('_fbp=')[1];
});
var fbc = document.cookie.split(';').filter(function (c) {
return c.includes('_fbc=');
}).map(function (c) {
return c.split('_fbc=')[1];
});
fbp = fbp.length && fbp[0] || null;
fbc = fbc.length && fbc[0] || null;
if(!fbc && window.location.search.includes('fbclid=')){
fbc = 'fb.1.'+ (+new Date()) +'.'+ window.location.search.split('fbclid=')[1];
}
var headers = new Headers();
headers.append("Content-Type", "application/json");
var body = {
"event": event,
"fbp": fbp,
"fbclid": fbc,
"user_agent": navigator.userAgent,
"url": window.location.origin + window.location.pathname
};
body = Object.assign({}, body, eventData)
var options = {
method: "POST",
headers: headers,
mode: "no-cors",
body: JSON.stringify(body)
};
fetch("ZAPIER_WEBHOOK_URL", options);
}
</script>
The only change you need to do in this code is to replace the ZAPIER_WEBHOOK_URL with, well, your Zapier webhook URL (see Step 1 above). Set the tag to trigger on All Pages.
Step 4 – Triggering the script
Once we have the script in place on all pages, we can now invoke it with the Facebook CAPI events we want to send.
These events can be invoked just like the regular Facebook events that use the fbq() method. Instead, they use the CAPI() method.
For example, in parallel to sending fbq(‘track’, ‘Lead’) you can send CAPI(‘Lead’). Notice that there’s no need to use the ‘track’ parameter.
You can also pass custom parameters, such as purchase value and currency, by invoking CAPI(‘Purchase’, {currency: “USD”, value: 30.00}). Since the data structure is identical to the Facebook Pixel, there’s little development effort required here (as the same triggers and data object apply here).
For example. you can use a Custom HTML tag that triggers on Purchase events with this code:
<script>
CAPI("Purchase",{currency:"USD",value:30.00)
</script>